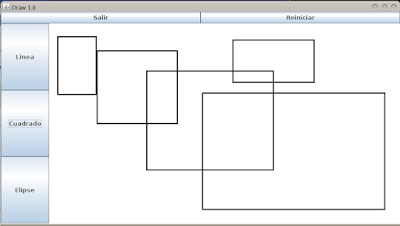
Hola a todos!!
Bueno pues esta vez les traigo esta pequena aplicacion que aun estoy perfeccionando.
Se trata de una aplicacion tipo Paint, pero tiene algunos bugs que debo corregir
BUGS:
- Hasta ahora solo tengo un bug reconocible, si ustedes ejecutan la aplicacion y dibujan lineas todo va bien, pero ya que se agregaron varias lineas al momento de querer dibujar cuadros, todos los objetos se vuelven cuadros, y al momento de querer agregar elipses todos los objetos se vuelven elipses x_x.
Se aceptan propuestas para arreglarlo e igual lo dejo posteado para que lo revisen y tomen lo que les sirva :)
OTROS OBJETIVOS
- Boton que permita controlar el grosor y color de linea
- Boton que permita dibujar figuras solidas
- Agregar mas figuras a la barra
Gracias por su visita ^_^
PD: Ya agregue links de descarga a todos mis codigos, se encuentran al final de cada entrada.
CODIGO:
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Iterator;
import java.awt.event.*;
class Linea {
protected double xs, ys, xf, yf;
public Linea(double x1, double y1, double x2, double y2) {
this.xs = x1;
this.ys = y1;
this.xf = x2;
this.yf = y2;
}
}
public class Paint extends JPanel implements MouseListener, MouseMotionListener, ActionListener {
public void actionPerformed(ActionEvent e) {
String cmd = e.getActionCommand();
if(cmd.equals("LINE")) {
this.linea = true;
this.cuadro = false;
this.circulo = false;
}
if(cmd.equals("SQUARE")) {
this.cuadro = true;
this.linea = false;
this.circulo = false;
}
if(cmd.equals("ELLIPSE")) {
this.circulo = true;
this.linea = false;
this.cuadro = false;
}
if (cmd.equals("EXIT")) {
System.exit(1);
} else if (cmd.equals("RESET")) {
this.lineas.clear();
this.repaint();
}
return;
}
public void mouseClicked(MouseEvent e) {
int ancho = super.getWidth();
int altura = super.getHeight();
double x = (e.getX() / (double)ancho);
double y = (e.getY() / (double)altura);
if (!this.dibujando) {
this.startX = x;
this.startY = y;
this.ahoraX = x;
this.ahoraY = y;
this.dibujando = true;
} else {
this.lineas.add(new Linea(this.startX, this.startY, x, y));
this.dibujando = false;
this.startX = Paint.INVALIDO;
this.startY = Paint.INVALIDO;
this.ahoraX = Paint.INVALIDO;
this.ahoraY = Paint.INVALIDO;
}
this.repaint();
return;
}
private Listlineas;
public void mouseEntered(MouseEvent e) {
return;
}
public void mouseExited(MouseEvent e) {
return;
}
public void mousePressed(MouseEvent e) {
return;
}
public void mouseReleased(MouseEvent e) {
return;
}
public void mouseDragged(MouseEvent e) {
return;
}
public void mouseMoved(MouseEvent e) {
if (this.dibujando) {
int ancho = super.getWidth();
int altura = super.getHeight();
double x = (e.getX() / (double)ancho);
double y = (e.getY() / (double)altura);
this.ahoraX = x;
this.ahoraY = y;
this.repaint();
}
return;
}
public static void main(String[] args) {
JFrame ventana = new JFrame();
ventana.setTitle("Draw 1.0");
ventana.setLocation(100, 100);
ventana.setSize(854, 480);
ventana.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Paint r = new Paint();
JPanel p1 = new JPanel();
p1.setLayout(new BorderLayout());
JPanel p2 = new JPanel();
p2.setLayout(new GridLayout());
JPanel p3 = new JPanel();
p3.setLayout(new GridLayout (3, 1));
JButton b1 = new JButton("Salir");
b1.addActionListener(r);
b1.setActionCommand("EXIT");
p2.add(b1);
p1.add(p2, BorderLayout.NORTH);
b1 = new JButton("Reiniciar");
b1.addActionListener(r);
b1.setActionCommand("RESET");
p2.add(b1);
p1.add(p2, BorderLayout.NORTH);
JButton b2 = new JButton("Linea");
b2.addActionListener(r);
b2.setActionCommand("LINE");
p3.add(b2);
p1.add(p3, BorderLayout.EAST);
b2 = new JButton("Cuadrado");
b2.addActionListener(r);
b2.setActionCommand("SQUARE");
p3.add(b2);
p1.add(p3, BorderLayout.EAST);
b2 = new JButton("Elipse");
b2.addActionListener(r);
b2.setActionCommand("ELLIPSE");
p3.add(b2);
p1.add(p3, BorderLayout.WEST);
p1.add(r, BorderLayout.CENTER);
ventana.setContentPane(p1);
ventana.setVisible(true);
return;
}
private static final BasicStroke TIPO = new BasicStroke(3.0f);
private static final Color ACTUAL = Color.RED;
private static final Color GUARDADO = Color.BLACK;
public void paint(Graphics gr) {
if(this.linea) {
super.paint(gr);
Graphics2D g = (Graphics2D)gr;
int ancho = super.getWidth();
int altura = super.getHeight();
g.setStroke(Paint.TIPO);
Iteratori = this.lineas.iterator();
while (i.hasNext()) {
Linea l = i.next();
g.draw(new Line2D.Double(l.xs*ancho,
l.ys*altura,
l.xf*ancho,
l.yf*altura));
}
if (this.dibujando) {
g.setColor(Paint.ACTUAL);
g.draw(new Line2D.Double(this.startX*ancho,
this.startY*altura,
this.ahoraX*ancho,
this.ahoraY*altura));
}
return;
}
if(this.cuadro) {
super.paint(gr);
Graphics2D g = (Graphics2D)gr;
int ancho = super.getWidth();
int altura = super.getHeight();
g.setStroke(Paint.TIPO);
Iteratori = this.lineas.iterator();
while (i.hasNext()) {
Linea l = i.next();
g.draw(new Rectangle2D.Double(l.xs*ancho,
l.ys*altura,
l.xf*ancho,
l.yf*altura));
}
if (this.dibujando) {
g.setColor(Paint.ACTUAL);
g.draw(new Rectangle2D.Double(this.startX*ancho,
this.startY*altura,
this.ahoraX*ancho,
this.ahoraY*altura));
}
return;
}
if(this.circulo) {
super.paint(gr);
Graphics2D g = (Graphics2D)gr;
int ancho = super.getWidth();
int altura = super.getHeight();
g.setStroke(Paint.TIPO);
Iteratori = this.lineas.iterator();
while (i.hasNext()) {
Linea l = i.next();
g.draw(new Ellipse2D.Double(l.xs*ancho,
l.ys*altura,
l.xf*ancho,
l.yf*altura));
}
if (this.dibujando) {
g.setColor(Paint.ACTUAL);
g.draw(new Ellipse2D.Double(this.startX*ancho,
this.startY*altura,
this.ahoraX*ancho,
this.ahoraY*altura));
}
return;
}
}
public Paint() {
super();
super.setBackground(Color.WHITE);
this.dibujando = false;
this.lineas = new ArrayList();
this.startX = Paint.INVALIDO;
this.startY = Paint.INVALIDO;
this.ahoraX = Paint.INVALIDO;
this.ahoraY = Paint.INVALIDO;
this.addMouseListener(this);
this.addMouseMotionListener(this);
}
private static final double INVALIDO = -1.0;
private boolean dibujando;
private boolean linea;
private boolean cuadro;
private boolean circulo;
private double startX;
private double startY;
private double ahoraX;
private double ahoraY;
}
DESCARGA: Paint.java